Coding is more than just writing lines of text; it is an art that combines logic, creativity, and discipline. For both novice and sage developers, there exist certain “unspoken rules” that separate average coding practices from exceptional ones. These rules are the bedrock of clean, maintainable, and efficient code, and understanding them is essential for anyone in the software development field, whether you’re just starting or have years of experience under your belt.
In this article, we’ll explore some of these unspoken rules and dive into how both novice and sage developers can apply them. Along the way, we’ll also look at some technical specifications and features to help you understand how these rules manifest in the real world.
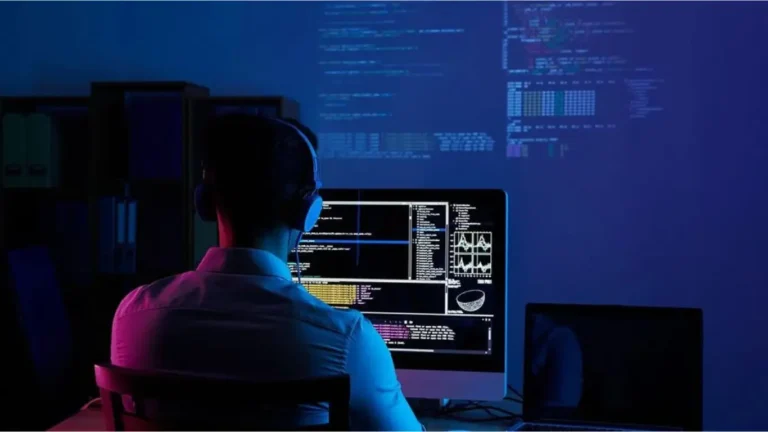
1. Write Code for Humans, Not Just Machines
One of the most critical principles in coding is that your code should be easy to read, understand, and maintain. While computers only care about whether the code works, other developers—whether it’s a colleague, future-you, or even an open-source community—will benefit from clear and concise code. This rule applies to both novice and seasoned developers alike.
The Game Archives Gameverse: A New Era in Gaming Exploration
Best Practices:
- Use meaningful variable names: For example, avoid naming a variable
x
when it represents a user’s age. A more descriptive name likeuserAge
orageInYears
makes it easier to understand. - Keep functions small: Break down complex tasks into smaller functions that do one thing well. This improves readability and debugging.
- Comment your code sparingly but effectively: Too many comments can clutter the code, while too few leave others in the dark. Provide comments where the code’s intent is not immediately obvious.
- Follow coding standards and style guides: Consistency is key. Use established guidelines (like PEP8 for Python or the Airbnb JavaScript Style Guide) to make your code readable and maintainable.
Example:
pythonCopyEdit# Poorly named and unclear code
def func(x, y):
return x + y
# Better, more descriptive version
def calculateSum(firstNumber, secondNumber):
return firstNumber + secondNumber
2. Embrace the Power of Refactoring
Refactoring is the process of restructuring your code without changing its external behavior. This might sound daunting, but it is an essential practice for keeping your codebase efficient and easier to maintain. Novice developers often overlook refactoring, while experienced developers understand its importance.
Best Practices:
- Don’t be afraid to revisit code: As your project grows, revisit old code and refactor it to simplify, optimize, and improve readability.
- Optimize only when necessary: Don’t prematurely optimize; focus on readability first, then optimize once you have measurable performance concerns.
- Use refactoring tools: Many IDEs (Integrated Development Environments) provide built-in tools to help you refactor code more efficiently. Take advantage of them.
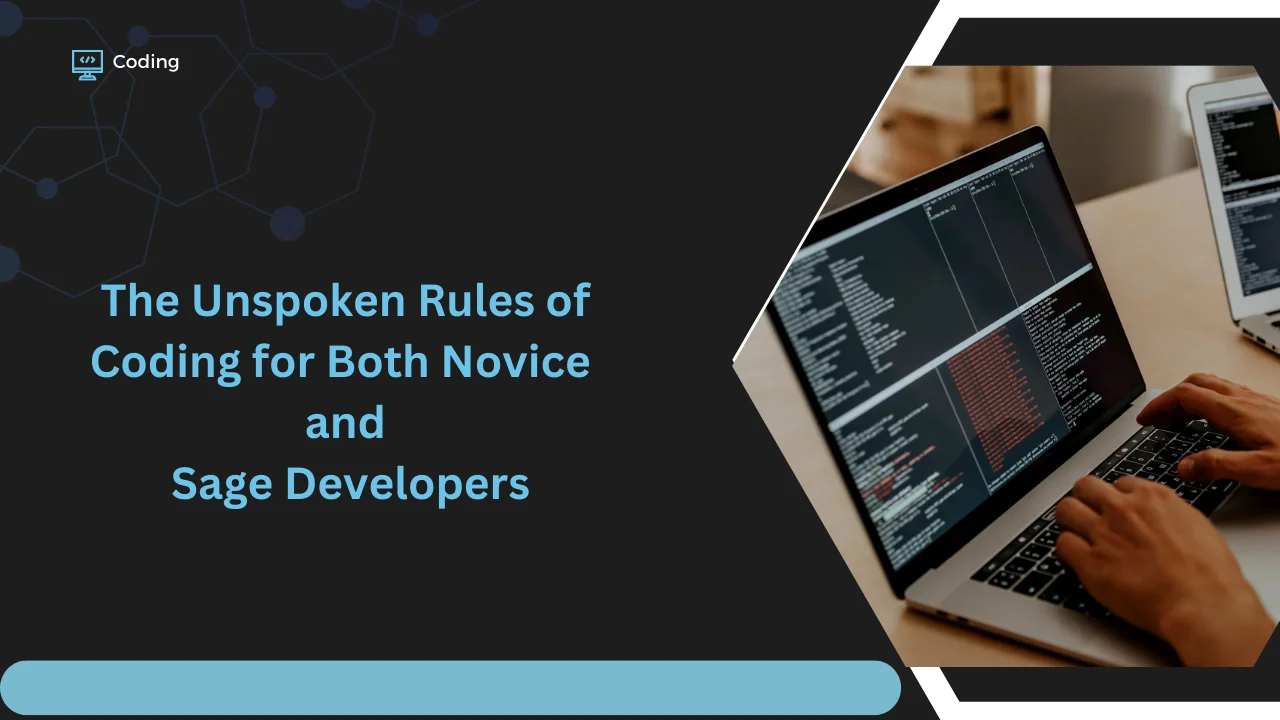
Example:
pythonCopyEdit# Before Refactoring
def get_user_data():
data = db.get_data('SELECT * FROM users WHERE active = 1')
user_list = []
for record in data:
user = {"name": record["name"], "email": record["email"]}
user_list.append(user)
return user_list
# After Refactoring
def get_active_users():
return db.get_data('SELECT name, email FROM users WHERE active = 1')
3. Always Test Your Code
Testing is one of the unspoken but crucial practices in coding. Whether you are a novice or a seasoned pro, testing ensures that your code behaves as expected and minimizes bugs in production. It’s better to write a test than assume your code is perfect.
Best Practices:
- Unit tests: Write tests for each function to check for correctness.
- Integration tests: Test the integration of various modules or services to ensure they work well together.
- Automate testing: Automate your tests with tools like Jenkins or GitHub Actions to ensure they run every time changes are made.
- Use TDD (Test-Driven Development): Write tests before writing the code. This helps to clarify what the code is supposed to do and prevents overcomplicating solutions.
Example:
pythonCopyEdit# Sample Unit Test for a simple function
def test_calculate_sum():
assert calculateSum(2, 3) == 5
assert calculateSum(-1, 1) == 0
4. Code Reviews Are Essential
Even the most experienced developers need a second set of eyes to review their code. Code reviews ensure that potential issues are caught early, improve the quality of the code, and offer an opportunity for collaborative learning.
Best Practices:
- Be open to feedback: Constructive criticism helps you grow as a developer, no matter your skill level.
- Review early and often: The sooner you catch bugs or bad practices, the easier they are to fix.
- Give meaningful feedback: Don’t just say “this doesn’t work.” Instead, suggest ways to improve or alternatives to consider.
Example:
Instead of saying:
“This part looks bad.”
Say:
“This part could be refactored for better readability. Perhaps consider breaking this function into two separate ones.”
5. Learn to Debug Effectively
Debugging is an art in itself. Understanding how to troubleshoot code and identify errors quickly is a skill every developer must master. For novices, debugging may seem frustrating, but with time and practice, it becomes an invaluable tool.
Best Practices:
- Use debugging tools: IDEs come with built-in debuggers that allow you to step through your code line by line, inspect variables, and track down the source of bugs.
- Understand the error message: Many novice developers tend to ignore error messages. However, error messages often contain vital clues to resolving issues.
- Add breakpoints: Set breakpoints to pause your code at specific locations and inspect the state at runtime.
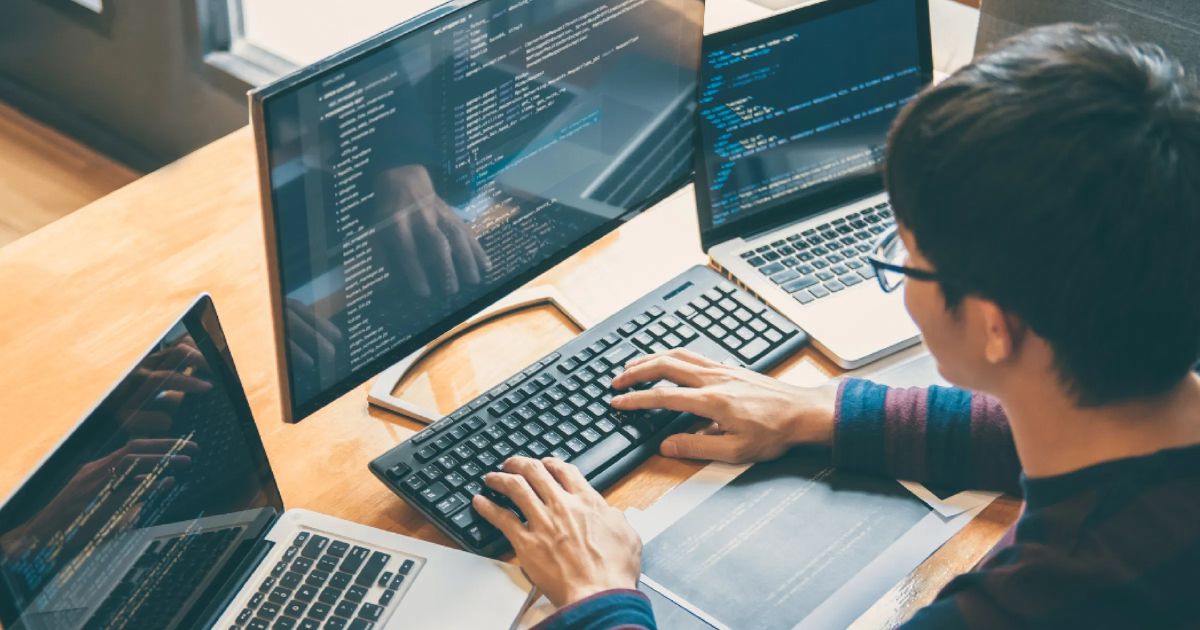
Example:
In Python, you can use the pdb
module to debug your code:
pythonCopyEditimport pdb
def faulty_function():
x = 10
y = 0
pdb.set_trace() # Pauses the execution
return x / y
6. Focus on Performance, But Don’t Obsess Over It
Performance is important, but premature optimization can lead to complicated code and wasted time. As a novice, it’s better to focus on writing clean, readable code first. Once your code is working, you can optimize it for performance if needed.
Best Practices:
- Avoid “over-engineering”: Don’t try to make your code overly complex just to gain a minor performance boost.
- Measure, don’t assume: Use profiling tools to measure performance bottlenecks rather than guessing where performance issues lie.
- Optimize the “hot spots”: Focus on optimizing the parts of the code that are used most frequently or impact performance the most.
Example:
pythonCopyEdit# Inefficient code
def calculate_fibonacci(n):
if n <= 1:
return n
return calculate_fibonacci(n-1) + calculate_fibonacci(n-2)
# Optimized version using memoization
def calculate_fibonacci(n, memo={}):
if n <= 1:
return n
if n not in memo:
memo[n] = calculate_fibonacci(n-1, memo) + calculate_fibonacci(n-2, memo)
return memo[n]
7. Documentation Is Your Friend
Well-documented code helps other developers understand how to use your work, as well as the reasoning behind certain decisions. Documentation should include not only code comments but also external documentation that explains your design choices, how to use your code, and what each function or class does.
Best Practices:
- Write clear README files: If you’re working on a project, always include a README with instructions on installation, usage, and examples.
- Document edge cases: If your code handles any edge cases, document these so that others understand the limitations and constraints of your solution.
Specs and Features Table
Feature | Novice Developer | Sage Developer |
---|---|---|
Code Readability | Often struggles with clear and concise code. | Prioritizes clear, easy-to-read code. |
Use of Comments | Comments code excessively or too little. | Comments only where necessary, keeping code clear. |
Refactoring | May neglect refactoring until later. | Frequently refactors code for clarity and efficiency. |
Testing | May skip tests due to lack of experience. | Uses comprehensive tests to ensure correctness. |
Debugging | Relies on trial and error. | Utilizes sophisticated debugging tools and methods. |
Performance Optimization | Focuses on working code rather than performance. | Optimizes after profiling and analysis. |
Code Reviews | Receives feedback reluctantly or sparsely. | Actively participates in reviews and gives constructive feedback. |
Documentation | Incomplete or vague documentation. | Provides comprehensive documentation for all code. |
Conclusion
Whether you’re just beginning your coding journey or you’ve been writing software for years, these unspoken rules are key to becoming a better, more efficient developer. By writing readable code, embracing testing and refactoring, and committing to continuous learning, both novice and sage developers can improve their craft and produce cleaner, more reliable software.
As you continue to develop your skills, always remember: coding is an ongoing learning process. Stay curious, stay open to feedback, and most importantly, enjoy the journey.